Fitting Service
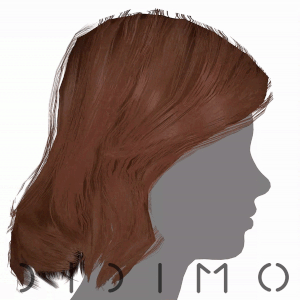
Character creation and customisation involves not only your base assets that define a didimo, but also additional assets such as hairstyles, beards, wearables, and so on. The possibilities are endless, and to make sure you can reuse these assets, we created the Fitting Service.
What is the Fitting Service?
The Fitting Service is provided through our API, and it deforms assets so they fit your didimos. Every time you create a didimo, you can request this service to deform your assets. It is composed of two parts, with one API call each:
Get
deformation matrix file for a given didimo. You should cache this file for subsequent Fitting API calls. Each didimo has its own deformation matrix file.Post
the asset you want to deform in.obj
format, along with the deformation matrix you got on step 1. Once deformation is done, you will be able to download a .zip file which contains the deformed asset, in.obj
format.
With this service, you'll be able to create your assets once, for the Base Mesh, and then reuse them in all your characters.
How do I use it?
For a quick start, our Unity SDK has an example implementation for deforming the hairstyles we provide. To use it, head over to the Generate a didimo guide. You will first create a didimo, and then add hairstyles and deform them.
For a more detailed guide, please read on.
Using the Fitting service with the Unity SDK
The Unity SDK has an integration of the Fitting Service
out of the box, so you do not need to know about the implementation details. You will simply need to follow these steps:
- Create your asset. It must fit our Base Template Mesh
- Import it into Unity. Create a prefab for it, give it a unique name, and configure everything you need (e.g. materials)
- Add the
Deformable
component to the prefab
3.1 Configure what joint of the didimo the asset should be parented to, by adding the joint name to theIdeal bone names
list - Add the prefab to the
Deformable Database
asset, located atAssets/Didimo/Core/Content/Resources/DeformableDatabase.asset
After this setup is complete, you are able to deform your assets. Here is a sample code snippet on how to do that:
async Task DeformAsset() {
didimoComponents.Deformables.TryCreate("ASSET_NAME", out Deformable deformable);
byte[] undeformedMeshData = deformable.GetUndeformedMeshData();
(bool success, byte[] deformedMeshData) = await Api.Instance.Deform(didimoComponents.DidimoKey, undeformedMeshData, progress => {
// TODO: Do something with 'progress'
});
if (!success) {
// TODO: Handle error case
return;
}
// Deform the mesh
deformable.SetDeformedMeshData(deformedMeshData);
}
Where ASSET_NAME
is the name of prefab you assigned in step 2.
If you need more customisation on how you handle your deformables, feel free to extend the Deformable
class. You can use the Hair
class as an example, as it is our implementation for didimo hairstyles that allows changing the hair color, among other things related to our hair assets.
Using the Fitting service without the Unity SDK
To deform your assets without using the Unity SDK, follow these steps:
- Create your asset. It must fit our Base Template Mesh
- Save it in
.obj
format, and make sure it conforms to the following requirements
2.1 Composed of geometric vertices only
2.2 Single mesh
2.3 Fits our Base Mesh
2.4 Root is at the origin
2.5 Units are in centimeters - Call our Get a Didimo by Its Key API endpoint, and download the file listed in
artifacts
with the namedeformer_dmx
. This file should be cached, so if you want to deform multiple assets, you don't need to requesting the file multiple times. - Call our Fit Assets API endpoint. The
user_asset
parameter is an.obj
file (ASCII encoded to bytes), and thetemplate_deformation
parameter is the file you downloaded on step 3. - Check the status of your fitting query, through one of the following options
5.1 Call the Get an Asset by Its Key API endpoint every few seconds until completion
5.2 Register to a webhook to be notified when the service is done processing - Download the asset deformed by our
Fitting Service
. The service will provide a .zip archive, and inside you can find your asset in.obj
format. Load it into your application anyway you see fit.
Updated about 2 years ago